How to Write Your First Java Application
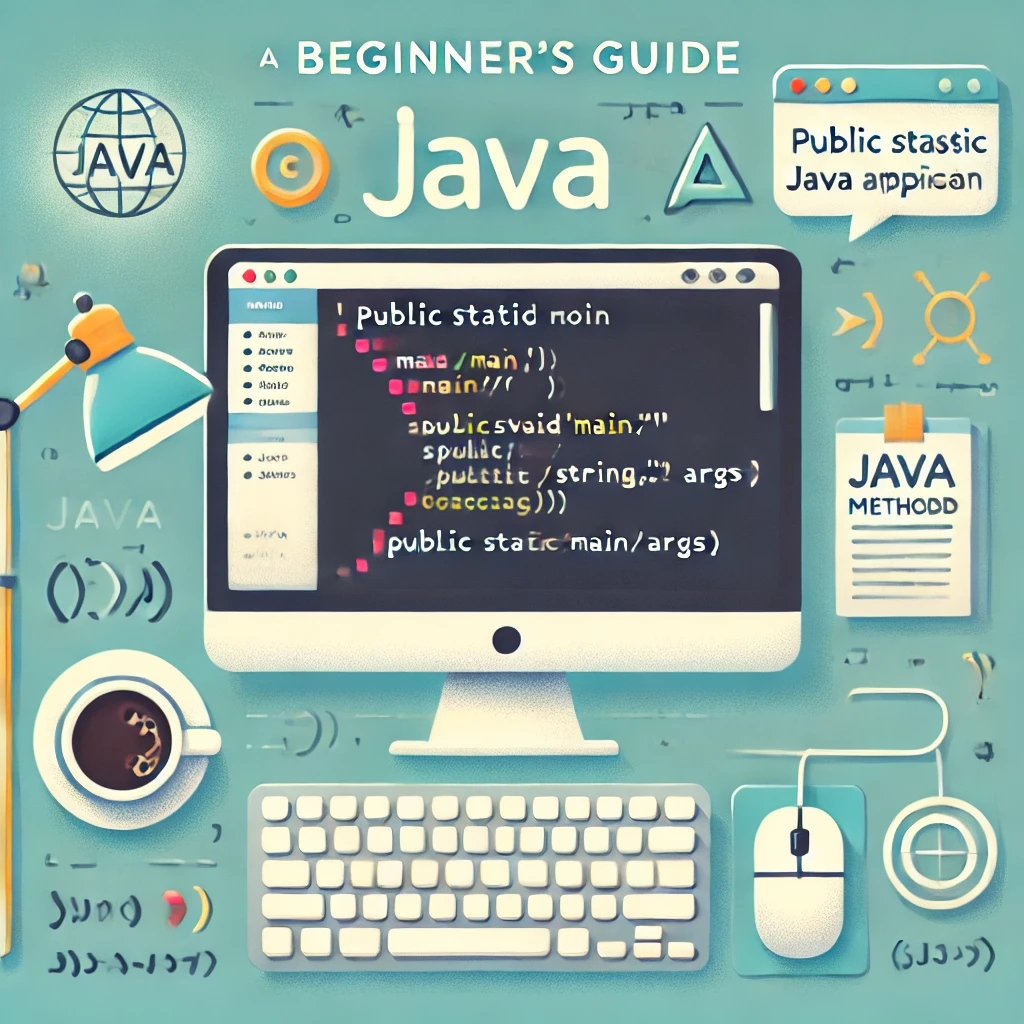
Example of the First Java Application
For Java development, specialized tools like IntelliJ IDEA are commonly used. We'll explore how to create a project in IntelliJ IDEA in a later lesson. However, as a beginner, you can write Java code in any text editor like Notepad or Notepad++. Just make sure to save the file with a .java
extension.
Here's an example of your first Java program in a file named MyFirstApp.java
:
public class MyFirstApp {
public static void main(String[] args) {
System.out.print("Hello world!!!");
}
}
Key Rules for Writing Java Applications
-
Source File Structure
A source file is the basic compilation unit. It contains definitions of one or more classes. For now, we'll stick to files with a single class. -
File Extension
The compiler requires source files to have the.java
extension. -
Class Naming
By convention, the main class name should match the filename. For example, if your file isMyFirstApp.java
, the class should be namedMyFirstApp
. -
All Code Must Reside in a Class
Java is class-based, so all code must be enclosed within a class. -
Case Sensitivity
Java is case-sensitive. Be consistent with uppercase and lowercase letters. -
Braces for Code Blocks
The class definition and its members must be enclosed in{}
curly braces. -
Entry Point: main() Method
Every Java application starts with amain()
method. Correct declarations include:public static void main(String[] args) static public void main(String[] args) public static void main(String... x) static public void main(String someArgs[])
While the compiler allows classes without amain()
method, such classes cannot execute without it. -
Method Parameters
Any required data for the method can be passed through parameters inside the parentheses. If no parameters are needed, use empty parentheses. -
Semicolons for Statements
Every statement should end with a semicolon (;
). -
Ignored Whitespace
Spaces, tabs, and newlines are generally ignored by the compiler, except where necessary for syntax.
By following these rules, you can confidently write, compile, and run basic Java applications.
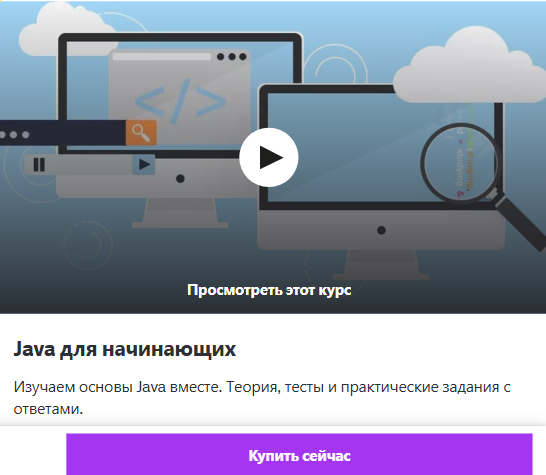
Please log in or register to have a possibility to add comment.