How to Use Command-Line Arguments in Java
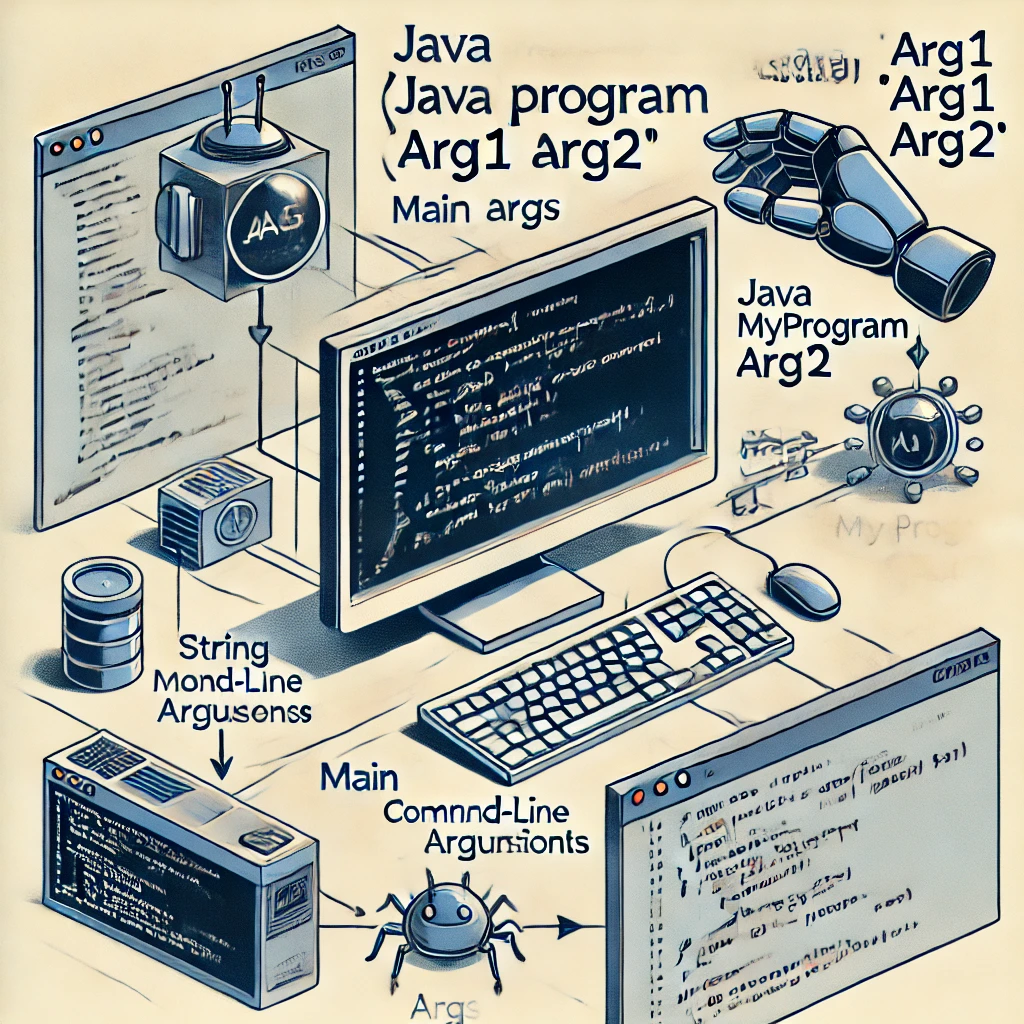
You can pass values to a program using command-line arguments. To run the MySecondApp
program and pass the values "value1" and "value2" as input, use the following command:
java com.company.lesson2.MySecondApp value1 value2
These values need to be received within the program. The main
method helps us achieve this:
public static void main(String[] args)
This method takes an array of type String
as a parameter: String[] args
. This variable will contain the values we passed: "value1" and "value2".
In the following example, the main()
method uses a for loop to iterate over the args
array and print the command-line arguments. We will discuss for loops and arrays in more detail in future lessons.
package com.company.lesson2;
public class MySecondApp {
public static void main(String[] args) {
for (String str : args) {
System.out.println("Argument = " + str);
}
}
}
Run the program using the following command:
java com.company.lesson2.MySecondApp value1 value2
Console output:
Argument = value1
Argument = value2
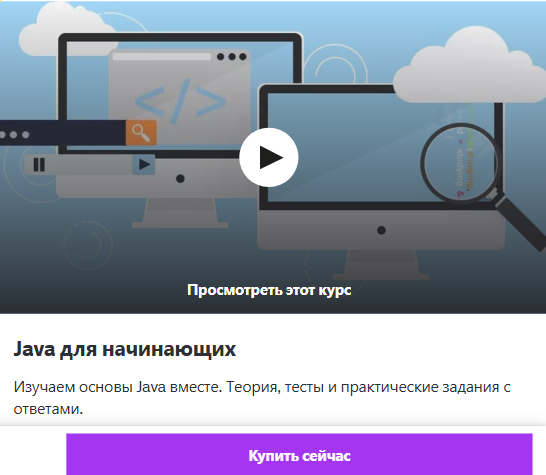
Please log in or register to have a possibility to add comment.