Understanding Java Compilation: -sourcepath and -classpath Explained
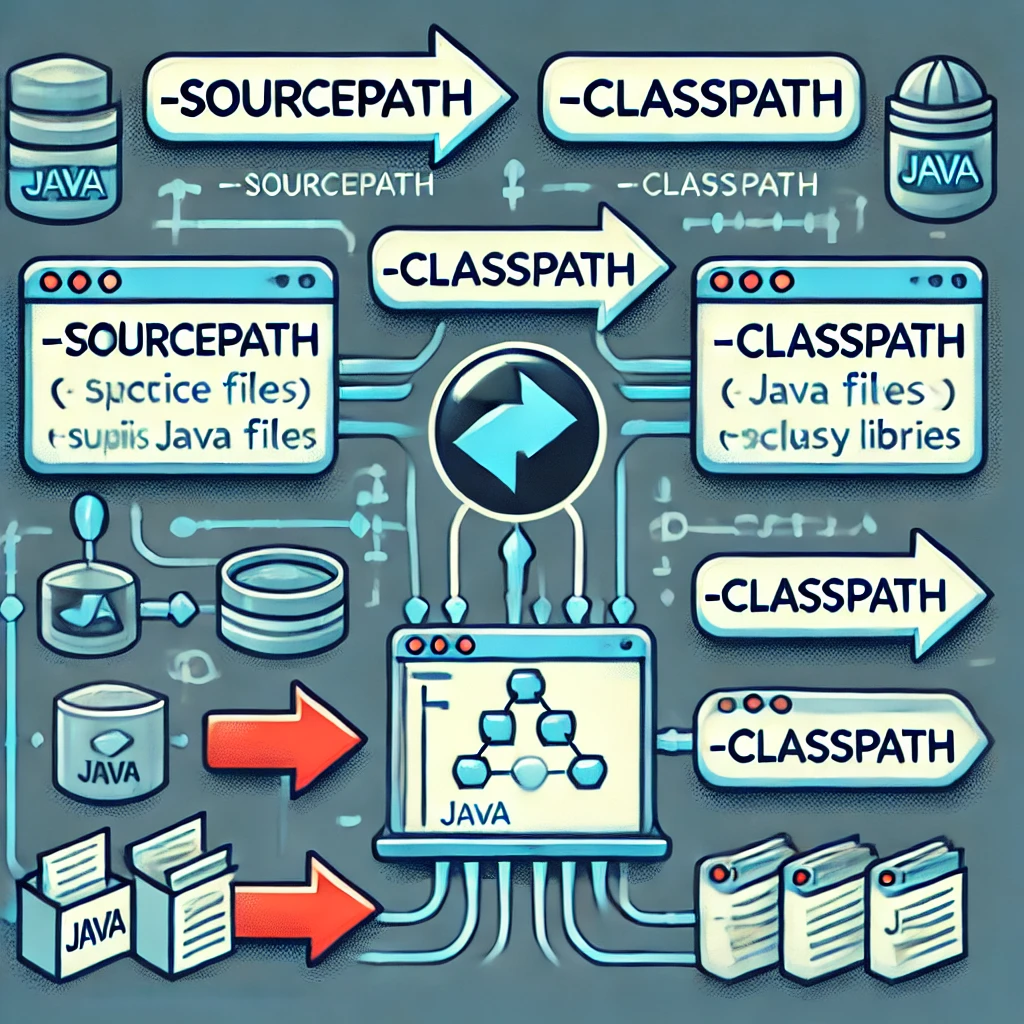
In this article, we will explore how to use the -sourcepath and -classpath options for compiling and running Java programs effectively.
1. Compilation with -sourcepath
The -sourcepath option specifies directories where the compiler should look for the source file hierarchy.
Consider an example with two classes in different packages: first.Example1
and second.Example2
. The first.Example1
class creates an instance of second.Example2
:
package first;
import second.Example2;
public class Example1 {
public static void main(String[] args) {
Example2 example2 = new Example2();
System.out.print("Done!");
}
}
package second;
public class Example2 {
}
Here is the directory structure:
Now, let's try compiling Example1.java
as we normally do:
cd project1
javac -d classes src/first/Example1.java
The compilation fails with the following errors:
src\first\Example1.java:9: error: package second does not exist
second.Example2 example2 = new second.Example2();
^
2 errors
This happens because the compiler doesn't know where to find Example2.java
. To fix this, use the -sourcepath option:
javac -d classes -sourcepath src src/first/Example1.java
2. What is Classpath?
The classpath is used by the java
and javac
commands to locate other classes needed during compilation and runtime. It can be specified as:
- An environment variable (CLASSPATH).
- The -classpath or -cp option in the
javac
andjava
commands, which overrides the environment variable for a specific call.
3. Using the -classpath Option
Suppose the second.Example2
class is in a different project and only its .class
files are available. Here's the directory structure:
To compile first.Example1
, use the following command:
cd projectExample1
javac -d classes -cp ../projectExample2/classes src/first/Example1.java
4. Key Points
- -sourcepath specifies directories for source files.
- -classpath (or -cp) specifies directories for compiled classes.
- Use relative or absolute paths for both options as needed.
- Including a subdirectory in the classpath doesn't automatically include the parent directory.
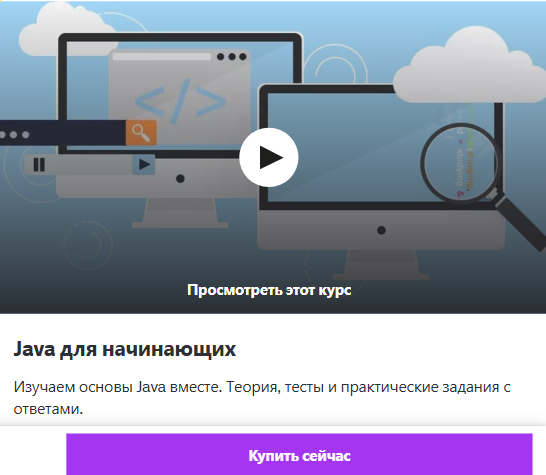
Please log in or register to have a possibility to add comment.