Primitive Type Conversion in Java: Implicit and Explicit Type Casting
In this lesson, we will talk about primitive type conversion in Java.
When does such conversion occur? Sometimes, there are situations where a variable of one type needs to be assigned a value of another type. For example:
int i = 11;
byte b = 22;
i = b;
Of course, in this case, type conversion occurs - the number from the byte type is converted to the int type. Conversely, a number of type int can be converted to byte:
int i = 11;
byte b = 22;
b = (byte)i;
Let's see what happens in both cases.
In the first case, we convert from byte to int, meaning from a smaller type to a larger type. This is a safe conversion that happens without issues. In binary form, it looks like this:
The byte value at the top is 8 bits. The int value at the bottom is 32 bits. The value in the int type is simply rewritten, and any missing values in the higher bits are replaced with zeros. This type of conversion is called automatic conversion.
But what happens in the second case when we do the reverse conversion from int to byte?
int i = 11;
byte b = 22;
b = (byte)i;
Here, we explicitly specify the conversion to byte. If we do not do this, a compilation error will occur. This conversion is not always safe. If a small number within the int range is converted, then the conversion will occur without issues. However, if we convert a number outside the byte range, such as 320, look at what happens:
The values that do not fit into the byte type are simply discarded, resulting in a different number. There is no rounding here. Converting 320 to byte results in 64.
This type of conversion is called type casting and is not safe. That is why we specify in parentheses that we are casting to byte, indicating to the compiler that we understand the potential data loss.
Thus, Java has two types of conversions - automatic conversion (implicit) and type casting (explicit conversion).
1. Automatic Type Conversion in Java
Let's first consider automatic conversion. If both types are compatible, Java performs the conversion automatically. For example, a byte
value can always be assigned to an int
variable, as shown in the previous example.
This conversion is safe and is also called implicit conversion because we do not explicitly specify that the number needs to be converted. Automatic conversion is also known as widening conversion because we expand our value from a smaller to a larger type.
Two conditions must be met for automatic conversion:
- Both types must be compatible
- The target type must be larger than the source type
In this case, widening conversion occurs.
But what are compatible types? For instance, all numeric types are compatible with each other. However, a boolean value and a numeric type are not compatible. Similarly, a string value like "Hello" cannot be converted to a numeric type.
The following diagram illustrates widening conversion in Java:
Solid lines indicate conversions performed without data loss. Dashed lines indicate that precision loss may occur during conversion.
2. Type Casting in Java
Despite the convenience of automatic type conversion, it cannot satisfy all needs. For example, what if an int
value needs to be assigned to a byte
variable? This conversion will not be performed automatically because the byte type is smaller than int. This type of conversion is sometimes called narrowing conversion because the value is explicitly narrowed to fit into the target data type.
To convert two incompatible data types, type casting is used. Type casting is simply an explicit type conversion. The general form of type casting is:
(target_type) value
Where target_type
specifies the type to which the given value should be converted.
This casting is also called explicit because we explicitly specify that we are casting to byte
or another type. It is not safe.
For example, in the following code snippet, an int
is cast to byte
:
int i = 11;
byte b = 22;
b = (byte) i;
3. Automatic Type Promotion in Expressions
Besides assignment operations, type conversion also occurs in expressions. For example, when adding two numbers—one of type int
and another of type byte
—what will the result be?
Java follows these rules:
- If one operand is
double
, the other is converted todouble
. - Otherwise, if one operand is
float
, the other is converted tofloat
. - Otherwise, if one operand is
long
, the other is converted tolong
. - Otherwise, both operands are converted to
int
. - In compound assignments (+=, -=, *=, /=), no explicit casting is needed.
For example:
byte b1 = 1;
byte b2 = 2 * b1; // Compilation error
int i1 = 2 * b1;
b2 *= 2;
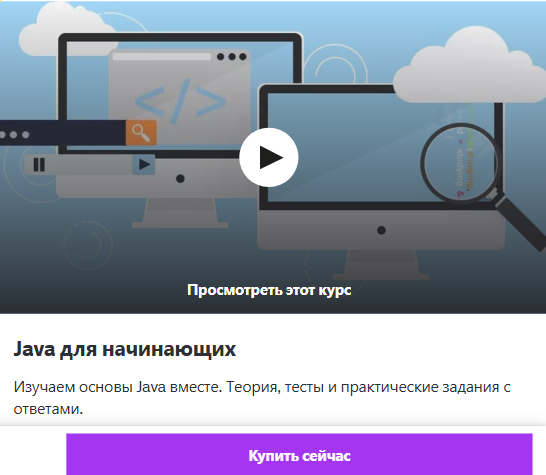
Please log in or register to have a possibility to add comment.