Java Methods: Syntax, Parameters, Return Types, and Best Practices
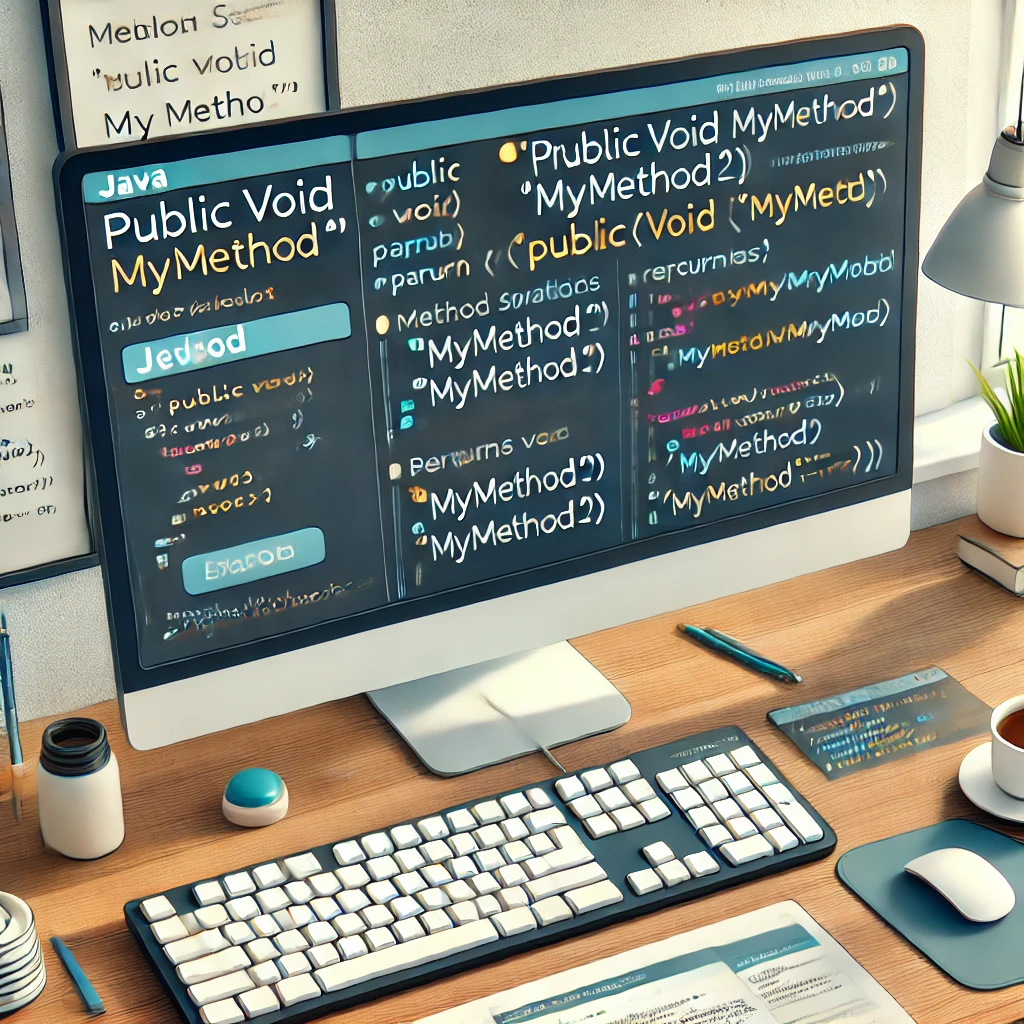
1. What is a Method in Java?
In today's lesson, we will take a detailed look at methods in Java programming. Almost all your Java code will be written inside methods, so understanding their syntax is essential. Let's start with the general form of a method declaration:
General method declaration:
type name(parameter_list) {
// method body
}
There is also a concept known as the Java method signature, which consists of the method name and its parameters. The return type is not part of the signature.
Each class can contain any number of methods.
Let's look at some examples.
Methods in Java That Do Not Return a Value
In the following example, the print
method does not accept any input values, meaning its parameter list is empty. Its return type is void
, which means it does not return anything.
The method prints the message "Print some info" to the console. The method declaration also includes the static
keyword. We will discuss its meaning in the Java Static Method lesson. For now, we will declare all methods with the static
keyword:
static void print() {
System.out.println("Print some info");
}
Methods in Java That Return a Value
The getVolume
method takes three parameters of type double
and returns a value of type double
. The method returns a value using the return
keyword:
static double getVolume(double width, double height, double depth) {
return width * height * depth;
}
2. Method Type
The type specifies the specific data type returned by the method. It can be any valid data type, including a user-defined class type.
If a method does not return a value, its return type must be void.
Methods that have a return type other than void
return a value using: return value;
3. Method Name and Parameters
The method name is represented by an identifier. It can be any valid identifier, except those already used by other code elements in the current scope.
The parameter list represents a sequence of "type-identifier" pairs separated by commas. Essentially, parameters are variables that receive values from arguments passed to the method during its call. If a method has no parameters, the parameter_list is empty.
4. Declaring and Calling a Java Method
Let's look at an example: we have a class SquareExample
that contains the main
method and a square
method. The square
method has a return type of int
and takes one parameter of type int
. The square
method simply multiplies an integer value by itself:
public class SquareExample {
public static void main(String[] args) {
int x, y;
x = square(5);
System.out.println(x);
x = square(9);
System.out.println(x);
y = 2;
x = square(y);
System.out.println(x);
}
public static int square(int i) {
return i * i;
}
}
When we define a method like this, we are only declaring it. However, it will not execute unless explicitly called. In the main
method, we see three calls to our square
method. The square
method requires a value compatible with the parameter int i
. This can be a literal or a variable.
When writing methods, we define one method after another. A method cannot be declared inside another method.
5. Parameter vs. Argument
It is important to distinguish between the terms parameter and argument.
A parameter is a variable defined within a method that receives a value when the method is called.
An argument is a value passed to a method during its call. For example, square(100)
passes 100
as an argument. Inside the square()
method, the parameter i
receives this value.
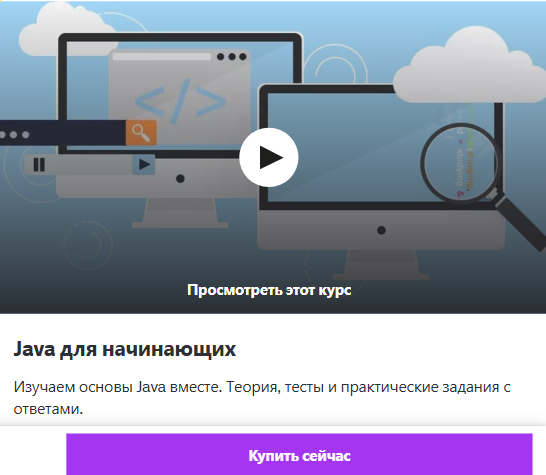
Please log in or register to have a possibility to add comment.