Essential Components of a Java Program
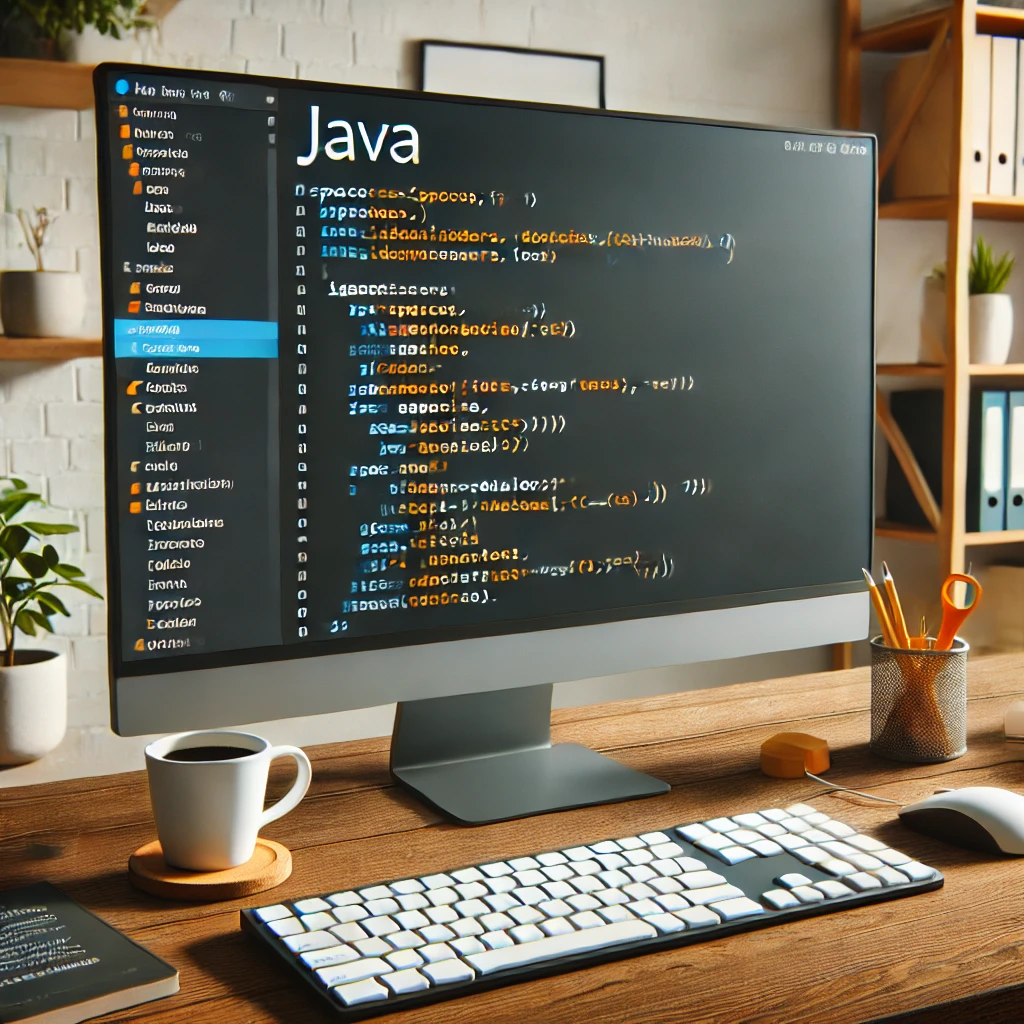
In today's lesson, we will start exploring the lexicon of the Java language and understand the components of a Java program.
1. Whitespace
Let's begin by examining whitespace.
Java is a free-form language, meaning there are no strict rules regarding whitespace when writing a program.
In Java, whitespace includes spaces, tabs, and newlines. You can insert multiple spaces, tabs, or line breaks, and the code will still compile. The only requirement is at least one space between lexemes that are not already separated by an operator or delimiter.
2. Identifiers
Next, let's discuss identifiers. What is an identifier? Identifiers are used to name classes, methods, or variables. For example, in the following program, MyFirstApp
is the class name (identifier), main
is a method identifier, and args
is also an identifier:
public class MyFirstApp {
public static void main(String[] args) {
System.out.println("Hello world!!!");
}
}
There are specific rules for choosing valid identifiers:
- An identifier can consist of letters (uppercase and lowercase), digits, underscores (_), and dollar signs ($).
- However, an identifier CANNOT start with a digit.
- Java is case-sensitive.
- Valid identifiers:
MinTemp
,sum
,x4
,$test
,my_number
. - Invalid identifiers:
3min
,min-temp
,yes/no
. - Keywords cannot be used as identifiers.
3. Keywords and Reserved Words
What are keywords? Java 23 defines 66 keywords and reserved words, which, together with syntax operators and delimiters, form the foundation of the Java language.
The following list includes all Java keywords:
- abstract
- assert
- boolean
- break
- byte
- case
- catch
- char
- class
- const
- continue
- default
- do
- double
- else
- enum
- exports
- extends
- final
- finally
- float
- for
- goto
- if
- implements
- import
- instanceof
- int
- interface
- long
- module
- native
- new
- package
- private
- protected
- public
- requires
- return
- short
- static
- strictfp
- super
- switch
- synchronized
- this
- throw
- throws
- transient
- try
- void
- volatile
- while
These words cannot be used as identifiers, or a compilation error will occur. You don’t need to memorize them; you will learn them throughout the course.
Reserved Words:
- true
- false
- null
These should also not be used as identifiers.
4. Comments
Comments are parts of the code ignored by the compiler, used to provide explanations and descriptions to make the code more readable. They help document functionality, logic, and key parts of the code.
There are three types of comments: single-line, multi-line, and documentation comments.
Single-Line Comments
In IntelliJ IDEA, commented-out code appears in gray:
// int avgTemp = 1;
Multi-Line Comments
You can comment out multiple lines of code using /* ... */
.
/* public static long roundTime(long time) {
return time / THOUSAND * THOUSAND;
}*/
Documentation Comments
These comments are typically used for classes or methods and can be converted into documentation.
/**
* Created by Tatyana on 07.04.2017.
*/
5. Delimiters
Java uses the following delimiters:
- Parentheses
( )
: Used for method parameters, type casting, and expressions. - Curly braces
{ }
: Define classes, methods, and code blocks. - Square brackets
[ ]
: Define arrays and access elements. - Semicolon
;
: Ends statements. - Comma
,
: Separates variable identifiers and constructs loops. - Dot
.
: Separates package names, sub-packages, classes, variables, and methods.
As you can see, delimiters are crucial for structuring Java programs.
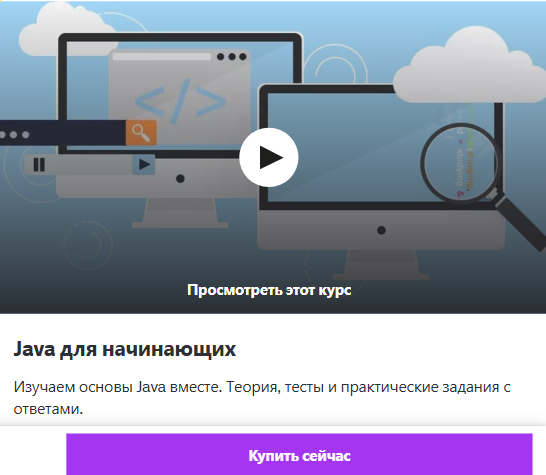
Please log in or register to have a possibility to add comment.