Java Variables: Declaration, Initialization and Scope
In this article, we will discuss Java variables in more detail.
1. Declaring and Initializing Variables in Java
Java variables are the fundamental storage elements in a Java program.
Before using a variable, it must be declared. We specify its type and name using an identifier, for example:
int max; // Declares the variable max
Here, we have only declared the variable max
, and its value is currently set to the default. For int
, this is zero. Later in the program, we can initialize the variable:
max = 89; // Initializes the variable max
It is generally better not to initialize it in the next line immediately.
If we need to declare multiple variables of the same type, we can do so in a comma-separated list:
int count = 0, min = 6; // Declares two integers: count and min
A variable can also be initialized during declaration:
double d = 2.3; // Declares and initializes the variable d
The initialization expression, meaning what we write after the equals sign, must return a value of the same or a compatible type as the declared variable. We will learn more about compatible types in our next lesson.
Java is a strongly typed language, meaning every Java variable has a specific type. The type of a variable determines:
- Its size and memory allocation,
- The range of values it can store,
- The set of operations that can be performed on it.
Once a variable is declared with a specific type, its type cannot be changed.
Of course, the value of a variable can change during program execution. Suppose we assign 8
to the variable max
and later modify it:
int max = 8;
...
max = 89;
2. Variable Scope in Java
In Java, variables can be declared within any code block. A code block is enclosed in curly braces, defining its scope or visibility. Each new block of code creates a new scope.
- The two main scopes in Java are defined by classes and methods, although this distinction is somewhat artificial.
- In Java, the visibility of a variable declared in a method starts from the opening curly brace of the method.
- Method parameters are included in the method’s scope and behave like any other variable in the method.
- Variables declared within a scope are not accessible outside that scope.
- Scopes can be nested.
- Variables are created when entering their scope and destroyed when exiting it.
- You cannot declare variables with the same name in an inner block if they exist in the outer block.
Let's examine variable scopes with an example:
public class VarExample {
String str1 = "Hello!"; // Variables str1 and str2 are declared in the class, meaning their scope is the class.
String str2 = "Hi!";
public static void main(String[] args) {
int x; // Variable x is accessible throughout the main() method
x = 10;
if (x == 10) { // Beginning of a new scope
int y = 20;
// int x = 45; // ERROR! A variable cannot be redeclared within an inner block
System.out.println("x and y: " + x + " " + y);
x = y * 2;
}
// y = 100; // ERROR! Variable y is not accessible here
System.out.println("x equals " + x);
}
}
3. Declaring Local Variables with var
Java 10 introduced the ability to declare local variables using the var keyword without specifying the type explicitly. The compiler infers the type from the assigned value:
public class VarExample2 {
public static void main(String[] args) {
var i = 6;
var d = 7.8;
var s = "some String";
System.out.println(i);
System.out.println(d);
System.out.println(s);
}
}
This type of declaration has its advantages and disadvantages.
The main advantage is that you do not need to specify the variable’s type explicitly. However, knowing the type of a variable helps understand what kind of value it holds. If you use var
, it is better to use meaningful variable names. For example, i
, d
, and s
are not very descriptive.
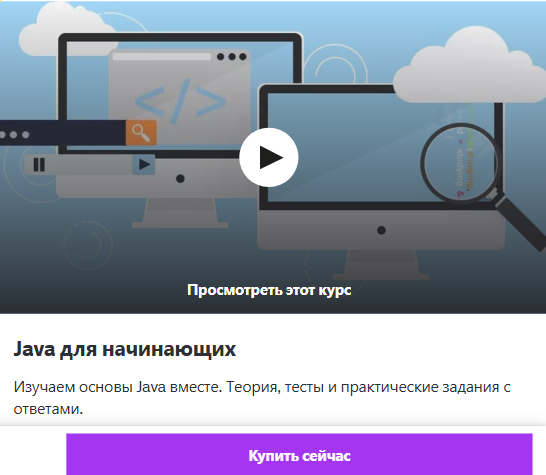
Please log in or register to have a possibility to add comment.