Java User Input: Reading Data from Keyboard with System.in and Scanner
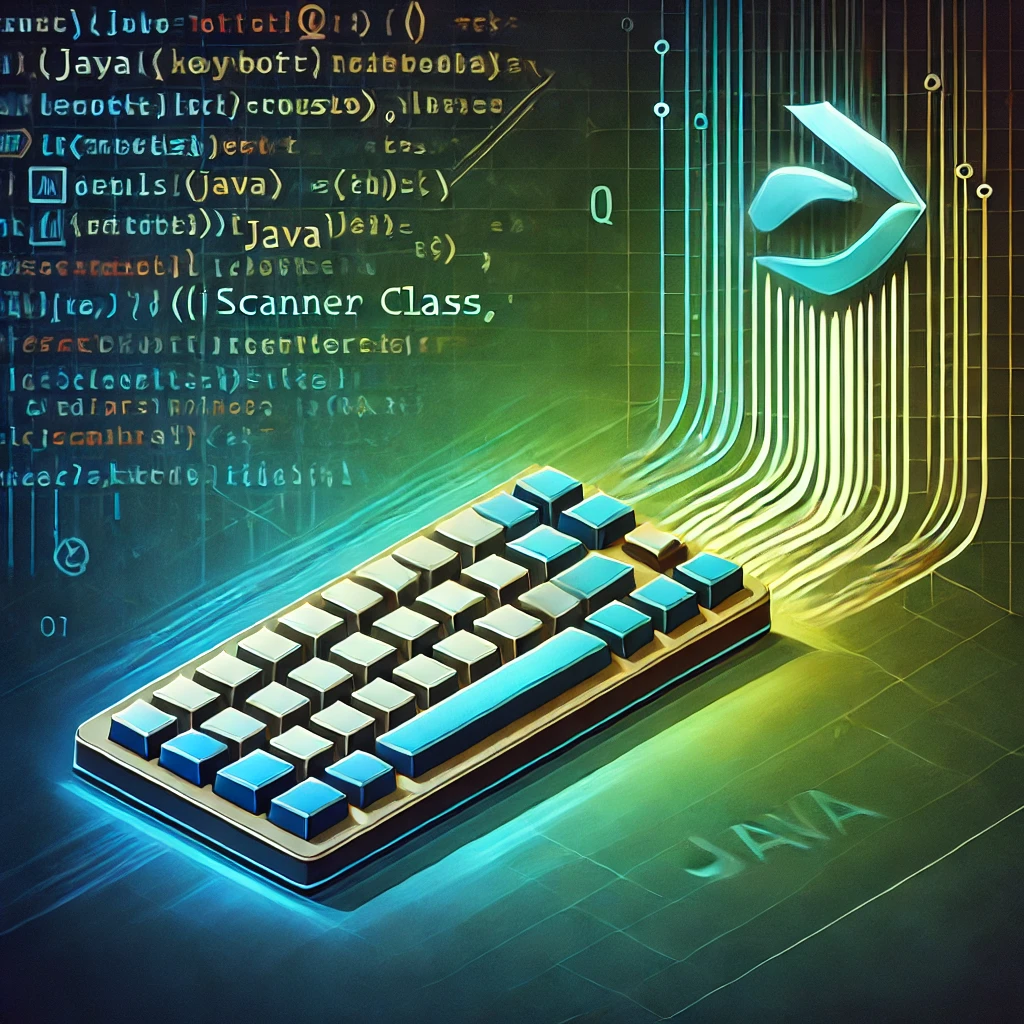
To allow users to enter input from the keyboard, Java provides a standard input stream, represented by the System.in object. Let's explore how it works.
For reading user input from the keyboard in Java, the System.in.read() method is used—it returns the code of the entered character. When executed, the JVM pauses the program and waits for the user to input a character. To display the character on the console, it is cast to the char type:
public class SystemInExample {
public static void main(String[] args) throws IOException {
int x = System.in.read();
char c = (char) x;
System.out.println("Character code: " + c + " = " + x);
}
}
Of course, using System.in directly is inconvenient when you need to input more than one character. In such cases, the Scanner class can be used. This class is located in the java.util package, so it must be imported:
import java.util.Scanner;
The methods of this class allow reading a string, an int
value, or a double
value.
Scanner Class Methods:
-
hasNextInt() - returns true if an integer can be read from the input stream.
-
nextInt() - reads an integer from the input stream.
-
hasNextDouble() - checks if a double-precision floating-point number can be read from the input stream.
-
nextDouble() - reads a double-precision floating-point number from the input stream.
-
nextLine() - reads an entire sequence of characters, i.e., a string.
-
hasNext() - checks if there are any characters left in the input stream.
In the following example, the hasNextInt() method checks if the user has entered an integer. If so, the nextInt() method reads the input. If the user enters a string, the program will output "You did not enter an integer" to the console:
import java.util.Scanner;
public class ScannerExample1 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter an integer: ");
if (scanner.hasNextInt()) {
int i = scanner.nextInt();
System.out.println(i);
} else {
System.out.println("You did not enter an integer");
}
}
}
Now, let's see an example using the nextDouble() method to read a floating-point number. If the user enters a string, the program will crash with a runtime error. To avoid this, check the input using hasNextDouble() before calling nextDouble():
import java.util.Scanner;
public class ScannerExample2 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// If you enter 's', a runtime error will occur
double i = scanner.nextDouble();
System.out.println(i);
}
}
The next example uses the nextLine() method to read an entire line of input:
import java.util.Scanner;
public class ScannerExample3 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String s1, s2;
s1 = scanner.nextLine();
s2 = scanner.nextLine();
System.out.println(s1 + s2);
}
}
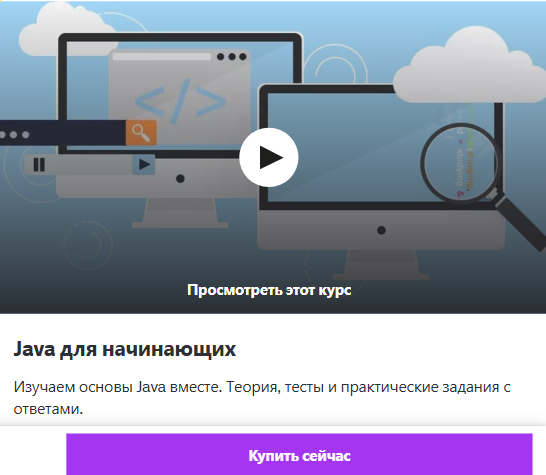
Please log in or register to have a possibility to add comment.