Operators
Welcome to the lesson on operations in Java! In this section, you will learn how to use various operators to perform calculations, comparisons, and other operations in your programs. We will cover the following key topics:
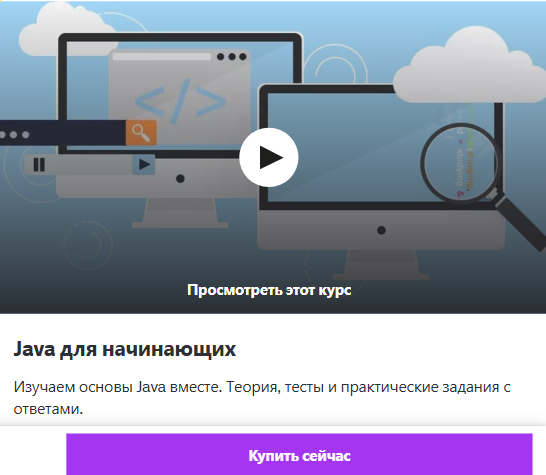
- Learn Java Arithmetic Operators and Compound Assignment with Examples
- Understanding Java Increment and Decrement Operators
- Understanding Java Comparison Operators: Equal, Greater, Less and More
- Java Logical Operators: Guide with Examples, Short-Circuit Evaluations
- Ternary Operator in Java – How It Works and When to Use It
- Mastering the Assignment Operator (=) in Java: Learn Its Syntax and Us
- Java Operator Precedence Table and Examples
- Java String Concatenation: Rules, Examples, and Unicode Encoding
Please log in or register to have a possibility to add comment.