Learn Java Arithmetic Operators and Compound Assignment with Examples
1. Basic Arithmetic Operators in Java
The following table lists the basic arithmetic operators (or operations) used in Java:
Operation | Description |
+ | Addition (also unary plus) |
- | Subtraction (also unary minus) |
* | Multiplication |
/ | Division |
% | Modulus (remainder of division) |
Here are some rules for working with arithmetic operators:
- Expressions are evaluated from left to right unless parentheses are used or some operations have higher precedence.
- The
*
,/
, and%
operations have higher precedence than+
and-
.
Arithmetic Operators with Integer Values
For example, in this code, variables a
and b
will have different values:
public class BasicIntMath {
public static void main(String[] args) {
int a = 4 + 5 - 2 * 3;
int b = 4 + (5 - 2) * 3;
System.out.println("a = " + a);
System.out.println("b = " + b);
}
}
Output:
a = 3
b = 13
Unary Addition and Subtraction Operations
- The unary subtraction operation changes the sign of its single operand.
- The unary addition operation simply returns the value of its operand. It is not necessary but is allowed.
public class UnarySignOperation {
public static void main(String[] args) {
double a = -6;
double b = +6;
System.out.println(a);
System.out.println(b);
}
}
Integer Division in Java
When division is performed on integer types, the result does not contain a fractional component. This is integer division in Java:
public class IntDivision {
public static void main(String[] args) {
int a = 16 / 5;
System.out.println(a);
}
}
Output:
3
Arithmetic Operators with char
Type Variables
Arithmetic operators require numeric types. They cannot be used with boolean data types, but they are allowed for char
types, as char
is essentially a subtype of int
in Java. For example:
public class BasicCharMath1 {
public static void main(String[] args) {
char c = 'n';
System.out.println(c);
System.out.println(c + 1);
System.out.println(c / 5);
}
}
Output:
n
111
22
Or in the following example:
public class BasicCharMath2 {
public static void main(String[] args) {
char c1 = '1';
char c2 = '\u0031';
char c3 = 49;
System.out.println(c1 + c2 + c3);
}
}
Output:
147
Modulus Division in Java
The modulus operator is represented by %
. It returns the remainder of division in Java. For integer division, the result is also an integer:
public class DivisionByModule {
public static void main(String[] args) {
int a = 6 % 5;
double b = 6.2 % 5.0;
System.out.println(a);
System.out.println(b);
}
}
Output:
1
1.2000000000000002
2. Compound Arithmetic Operations with Assignment
Operation | Description |
+= | Addition with assignment |
-= | Subtraction with assignment |
*= | Multiplication with assignment |
/= | Division with assignment |
%= | Modulus with assignment |
Java provides special operations that combine arithmetic operators with assignment. Consider the following expression:
a = a + 4;
In Java, this operation can be written as:
a += 4;
Compound assignment operations not only reduce code length but also perform automatic type conversion, which regular operations do not:
public class CompoundOperations {
public static void main(String[] args) {
int a = 1;
int b = 2;
int c = 3;
a += 3;
b *= 2;
c += a * b;
System.out.println(a);
System.out.println(b);
System.out.println(c);
}
}
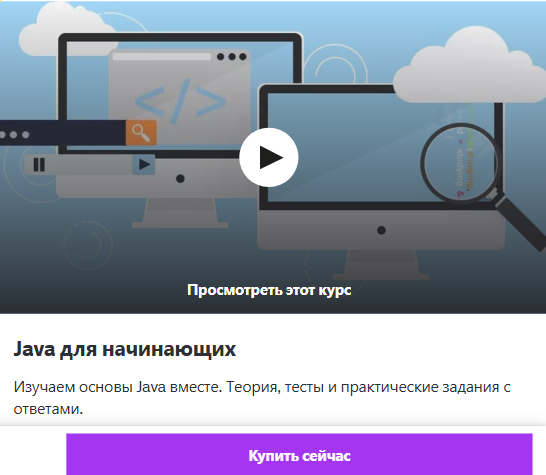
Please log in or register to have a possibility to add comment.