Understanding Java Increment and Decrement Operators
The ++
and --
operators perform increment and decrement. The increment operation increases the operand value by one, while the decrement operation decreases it by one. For example, the expression:
x = x + 1;
can be rewritten using the increment operator as:
x++;
Similarly, the following expression:
x = x - 1;
is equivalent to:
x--;
These operations can be written in postfix form, where the operation follows the operand, or in prefix form, where the operation precedes the operand.
Let’s examine the difference between prefix and postfix increment in Java using the following example. The difference appears only when the increment is used in another operation.
For instance, in the statement int b = a++;
, the value of a
is first assigned to b
, and only then does the increment take place (postfix form means "after"). As a result, the value of b
will be 1. Now, consider the next statement - int c = ++a;
- here, the increment happens first (prefix form means "before"), and only then is the new value of a
assigned to c
.
When the increment is not part of another operation, like in the statement c++
, it does not matter whether you use the prefix or postfix form.
public class IncrementDecrement {
public static void main(String[] args) {
int a = 1;
int b = a++;
int c = ++a;
c++;
System.out.println("a = " + a);
System.out.println("b = " + b);
System.out.println("c = " + c);
}
}
Program output:
a = 3
b = 1
c = 4
The increment operation can also be applied to variables of type char
. In this case, the increment is performed on the character's Unicode code. Since characters are ordered alphabetically in the Unicode table, applying the increment operator to a character value results in the next character in alphabetical order:
public class CharInc {
public static void main(String[] args) {
char ch = 'X';
System.out.println("ch contains " + ch);
ch++; // incrementing ch
System.out.println("New value of ch: " + ch);
}
}
Program output:
ch contains X
New value of ch: Y
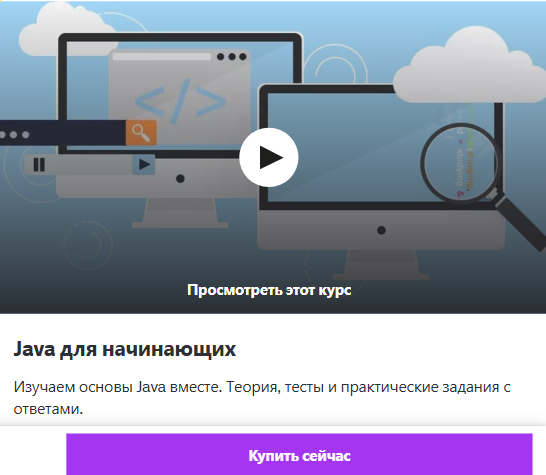
Please log in or register to have a possibility to add comment.