Java Logical Operators: Guide with Examples, Short-Circuit Evaluations
Java logical operators work only with operands of type boolean.
The following table lists the Java logical operators:
Operation | Description |
& | Logical AND operation (AND) or conjunction |
| | Logical OR operation (OR) or disjunction |
^ | Logical XOR operation (XOR) |
! | Logical NOT operation (NOT) |
|| | Short-circuit logical OR |
&& | Short-circuit logical AND |
== | Equality |
!= | Inequality |
&= | Logical AND with assignment |
|= | Logical OR with assignment |
^= | Logical XOR with assignment |
1. Logical Operators OR, AND, XOR, NOT
Let's start with the OR (|), AND (&), XOR (^), and NOT (!) operations. OR, AND, and XOR are binary operators, meaning they require two operands. NOT is a unary operator, meaning it operates on a single operand. The results of these logical operations are presented in the following table:
A | B | A | B | A & B | A ^ B | !A |
false | false | false | false | false | true |
true | false | true | false | true | false |
false | true | true | false | true | true |
true | true | true | true | false | false |
OR (|) - The result is true
if at least one of the operands is true
. Example: If either the mother or the father (or both) pick up the child from daycare, the result is positive. If neither comes, the result is negative.
AND (&) - The result is true
only if both A and B are true
. Example: For a wedding to take place, both the bride (A) and groom (B) must be present.
XOR (^) - The result is true
only if either A is true
or B is true
, but not both. Example: Two friends share one bicycle, and only one can ride at a time.
NOT (!) - Inverts the value. If the value was true
, it becomes false
, and vice versa.
Example of logical operators in Java:
public class BooleanLogic1 {
public static void main(String[] args) {
boolean a = true;
boolean b = false;
boolean c = a | b;
boolean d = a & b;
boolean e = a ^ b;
boolean f = (!a & b) | (a & !b);
boolean g = !a;
System.out.println("a = " + a);
System.out.println("b = " + b);
System.out.println("a | b = " + c);
System.out.println("a & b = " + d);
System.out.println("a ^ b = " + e);
System.out.println("(!a & b) | (a & !b) = " + f);
System.out.println("!a = " + g);
}
}
2. Short-Circuit Logical Operators
Java often uses short-circuit logical operators:
In short-circuit operations, the right operand is evaluated only if necessary. That is, if the left operand of an AND operation is false
, or the left operand of an OR operation is true
, the right operand is not evaluated.
Example demonstrating short-circuiting in Java:
public class BooleanLogic2 {
public static void main(String[] args) {
int d = 0;
int num = 10;
if (d != 0 && num / d > 10) {
System.out.println("num = " + num);
}
}
}
3. Equality and Inequality Operators
To compare boolean values, Java provides ==
(equality) and !=
(inequality) operators:
public class BooleanLogic4 {
public static void main(String[] args) {
boolean b1 = true;
boolean b2 = false;
System.out.println(b1 == b2);
System.out.println(b1 != b2);
}
}
4. Assignment Operations
Java also supports assignment operations for AND, OR, and XOR:
public class BooleanLogic5 {
public static void main(String[] args) {
boolean b1 = true;
boolean b2 = true;
b1 &= b2;
System.out.println(b1);
b1 |= b2;
System.out.println(b1);
b1 ^= b2;
System.out.println(b1);
}
}
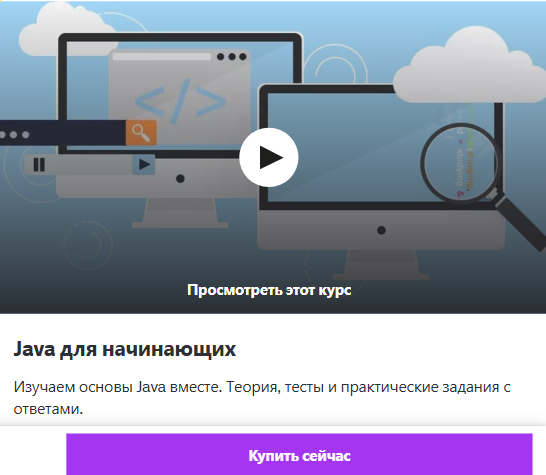
Please log in or register to have a possibility to add comment.