Java String Concatenation: Rules, Examples, and Unicode Encoding
String Concatenation (joining) is the operation of combining strings. In Java, this operation is performed using the +
operator. You can concatenate not only another string but also a value of any other type, which will be automatically converted to a string. How can you determine when the +
sign is concatenation and when it is an arithmetic operation?
There are only two rules to remember:
- If one of the operands is of String type, the
+
operator is used as string concatenation. - If both operands are numbers, the
+
operator is used for addition.
In this example, we simply concatenate two strings:
public class StringExample1 {
public static void main(String[] args) {
String str1 = "world";
System.out.println("Hello " + str1);
System.out.println("First line\n" + "Second line");
}
}
In the next example, the first System.out.println
statement will output "X Y" to the console. We concatenate a char
with a String
—this is string concatenation. However, in the second System.out.println
statement, the output will not be a string, as many expect, but the number 177. Why a number? The char type is a pseudo-integer type and can participate in arithmetic operations. In this case, the character codes are added:
public class StringExample2 {
public static void main(String[] args) {
char x, y;
x = 88; // Character code for 'X'
y = 'Y';
System.out.println(x + " " + y); // Outputs X Y
System.out.println(x + y); // Outputs 177
}
}
In the next example, the output will be "str=48"—don’t forget about operator precedence. The multiplication operation has a higher precedence than addition:
public class StringExample3 {
public static void main(String[] args) {
System.out.println("str=" + 4 + 4 * 2);
}
}
Unicode encoding can also be used in strings, just like in char
type variables:
public class StringExample4 {
public static void main(String[] args) {
// The word "Ukraine" in Unicode encoding
System.out.println("\u0423\u043A\u0440\u0430\u0438\u043D\u0430");
}
}
To compare two strings for equality, use the equals method or Objects.equals()
(starting from Java 7):
import java.util.Objects;
public class StringExample5 {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "Hi";
System.out.println(str1.equals(str2));
System.out.println(Objects.equals(str1, str2));
}
}
The length of a string is determined using the length()
method:
public class StringExample6 {
public static void main(String[] args) {
String str = "Hello";
System.out.println("String length: " + str.length());
}
}
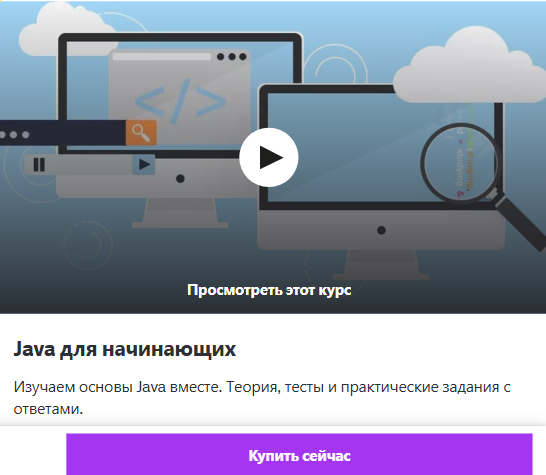
Please log in or register to have a possibility to add comment.