Sub-Resources
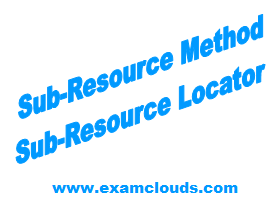
1. The Sub-Resource Methods
The sub-resource methods are methods of a resource class that are annotated with @Path and one of the request method designators (@GET, @PUT, @POST or @DELETE).
Example 1.1. Sub-resource Method
The getCategories() method is a sub-resource method, which is invoked by /exams/categories URI. If the request path is /exams, then resource method getExams() is invoked.
@Path("exams")
public class ExamService {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String getExams() {
...
}
@GET
@Path("/categories")
@Produces(MediaType.TEXT_PLAIN)
public String getCategories() {
...
}
}
2. The Sub-resource locators
Sub-resource locators are methods that are annotated with @Path but without a request method designator. Sub-resource methods handle an HTTP request directly, and sub-resource locators return an object that will handle an HTTP request.
- Sub-resource locators can reuse resource classes.
- A sub-resource locator supports polymorphism.
- The runtime doesn't manage the life-cycle or perform any field injection onto instances returned from sub-resource locator methods.
- The subresource locator can also return a programmatic resource model.
Example 2.1. Sub-resource Locator
The root resource class ExamService has the sub-resource locator method getCategories() which returns a new resource class. If the path of the request URL is "exams/categories" then first the root resource will be matched, then the sub-resource locator will be matched and called.
@Path("exams")
public class ExamService {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String getExams() {
...
}
@Path("/categories")
public CategoryService getCategories() {
return new CategoryService();
}
}
public class CategoryService {
@GET
public String getCategories() {
...
}
}
Example 2.2. Sub-resource Locators created from classes
If it is required that the runtime manages the sub-resources as standard resources the Class should be returned:
@Path("exams")
public class ExamService {
@Path("/")
public Class<CategoryService> getCategories() {
return CategoryService.class;
}
}
@Singleton
public class CategoryService {
@GET
public String getCategories() {
...
}
}
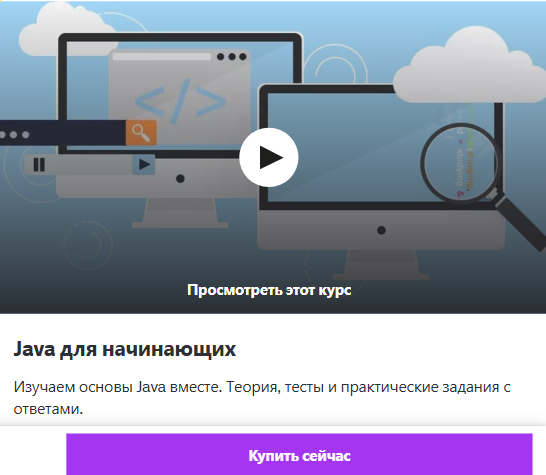
Please log in or register to have a possibility to add comment.