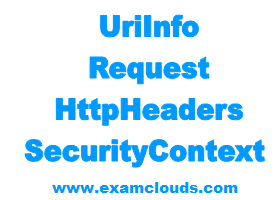
Use of @Context
JAX-RS gives the possibility to receive and process the application deployment context and the context of individual requests. An instance of UriInfo, HttpHeaders, Request, and SecurityContext can be injected into a class field or method parameter using the @Context annotation.
1. UriInfo
The UriInfo object gives the possibility to find or build URI information of a request. It can be used to obtain URIs and associated UriBuilder instances for the base URI the application is deployed at, the request URI, and the absolute path URI, which is the request URI minus any query components.
Example 1.1. Receive a map of URI path and query parameters
The example shows how to return the names of query and path parameters in a request. The UriInfo is injected into the method.
@GET
public String get(@Context UriInfo uriInfo) {
MultivaluedMap<String, String> queryParams = uriInfo.getQueryParameters();
MultivaluedMap<String, String> pathParams = uriInfo.getPathParameters();
...
}
Example 1.2. Receive absolute path of the resource
The method get() returns the absolute path of the resource. The UriInfo is injected into the class field, which is useful when it is impossible to change the method signature.
@Path("exams")
public class ExamService{
@Context
UriInfo uriInfo;
@GET
public String get() {
return uriInfo.getAbsolutePath().toASCIIString();
}
}
Example 1.3. Use UriBuilder for creating new URI
JAX-RS has the UriBuilder class which simplifies the process of building new URIs. In the example, UriBuilder is used to build from the template.
@Path("exams")
public class ExamService {
@Context
UriInfo uriInfo;
@GET
public String get() {
UriBuilder uriBuilder = uriInfo.getAbsolutePathBuilder();
URI newUri = uriBuilder.
path("{a}").
queryParam("type", "{b}").
build("categories", "json");
return newUri.toString();
}
}
2. HttpHeaders
The HttpHeaders provides access to request header information.
Example 2.1. Receive a general map of header parameters
It is possible to receive headers and cookies parameters with the help of the HttpHeaders.
@GET
public String get(@Context HttpHeaders httpHeaders) {
MultivaluedMap<String, String> headerParams = httpHeaders.getRequestHeaders();
Map<String, Cookie> cookies = httpHeaders.getCookies();
...
}
3. SecurityContext
The SecurityContext provides security information of the current request, for example, the current user principal, information about roles assumed by the requester, whether the request arrived over a secure channel, and the authentication scheme used.
Example 3.1. Accessing SecurityContext
@GET
public String get(@Context SecurityContext securityContext) {
if (securityContext.isUserInRole("sysadmin")) {
return "Sysadmin user";
} else {
return "Custom user";
}
}
4. Request
The usage of Request is covered in Building Responses.
Зарегистрируйтесь или войдите, чтобы иметь возможность оставить комментарий.