JAX-RS Parameter Annotations
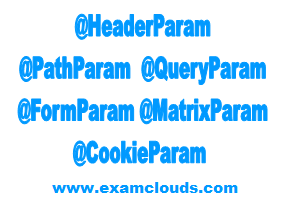
Parameter annotations are used with method parameters to get information from the request:
- @PathParam
- @QueryParam
- @HeaderParam
- @MatrixParam
- @CookieParam
- @FormParam
Requirements for Java type of the method parameter:
- Be a primitive type.
- Have a constructor that accepts a single String argument.
- Have a static method named valueOf or fromString which accepts a single String argument.
- Have a registered implementation of javax.ws.rs.ext. ParamConverterProvider JAX-RS extension SPI that returns a javax.ws.rs.ext. ParamConverter instance is capable of a "from string" conversion for the type.
- Be List<T>, Set<T> or SortedSet<T>, where T satisfies 2 or 3 above. The resulting collection is read-only. Collections are used when parameters may contain more than one value for the same name.
1. @PathParam
@PathParam is used to extract a path parameter.
Example 1.1. @PathParam Parameter
The method getExam() of the example will be called for the URI /exams/1.
@Path("exams")
public class ExamService {
...
@GET
@Path("/{id}")
@Produces(MediaType.TEXT_PLAIN)
public String getExam(@PathParam("id") int id) {
...
}
...
}
2. @QueryParam
@QueryParam extracts query parameters from the request.
Example 2.1. Query Parameters
Let's imagine we want not only to receive the full list of exams but specify the maximum number of received lists and the type of exams (public or private).
@GET
@Produces(MediaType.TEXT_PLAIN)
public String getExams(
@DefaultValue("3") @QueryParam("max") int max,
@DefaultValue("true") @QueryParam("public") boolean isPublic) {
...
}
- Query parameters "max" and "public" will be extracted and set to method variables "max" and "isPublic".
- If query parameters don't exist in the request, variables are set to values from @DefaultValue annotations.
- If @DefaultValue isn't set and the query parameter doesn't exist in request, then the value will be an empty collection for List, Set, or SortedSet and the Java-defined default for other types.
- If the "max" value cannot be transformed to an int then an HTTP 404 (Not Found) response is returned.
3. @HeaderParam and @CookieParam
@HeaderParam extracts information from the HTTP headers. @CookieParam extracts information from the cookies declared in cookie-related HTTP headers.
Example 3.1. Using @HeaderParam
@Path("exams")
public class ExamService {
@GET
public String getData(@HeaderParam("Referer") String referer) {
...
}
}
4. @MatrixParam
@MatrixParam extracts information from URL path segments, where ";" is used as a delimiter instead of "?".
Example 4.1. Matrix Parameter
For example, it is possible to extract the "max" parameter out of this URI: /exams;max=3.
@Path("exams")
public class ExamService {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String getExam(@MatrixParam("max") int max) {
...
}
}
5. @FormParam
@FormParam extracts information from the POSTed HTML forms. Request representation should have MIME media type "application/x-www-form-urlencoded" and conforms to the encoding specified by HTML forms. The next two examples demonstrate how to extract data from the posted HTML forms.
Example 5.1. Receive one Form Parameter
@POST
@Consumes("application/x-www-form-urlencoded")
public void addExam(@FormParam("name") String name) {
...
}
Example 5.2. Receive multiple Form Parameters
@POST
@Consumes("application/x-www-form-urlencoded")
public void addExam(MultivaluedMap<String, String> formParams) {
...
}
6. Injection Rules
Values can be injected on constructor parameters, fields, resource/sub-resource/sub-resource locator method parameters, and bean setter methods.
- Constructor parameters. The constructor will be called with injected values. If there are several constructors, the constructor with the most injectable parameters will be called.
- Class fields. Inject value into the field of the class. The field can be private and must not be final.
- Resource and sub-resource methods, sub-resource locators. They can contain parameters that are injected when the resource method is executed.
- Setter methods. The value can be injected into the setter method instead of the field. This type of injection can be used only with @Context annotation for injecting request. The setter method is called after the object creation and only once. The name of the method does not necessarily have a setter pattern.
Example 6.1. Injection to Root Resource
@Path("exams")
public class ExamResource {
@QueryParam("query1")
private String param;
public ExamResource(@QueryParam("query2") String queryParam) {
...
}
@GET
public String get(@QueryParam("query3") String queryParam) {
...
}
}
There are some restrictions when injecting to resource classes with a life-cycle of singleton scope. It is impossible to inject on the class fields, constructor parameters, and setter methods in such cases. But you can inject HttpHeaders, Request, UriInfo, SecurityContext request objects using the @Context annotation for these special cases.
The @FormParam annotation can be used only on resource and sub-resource methods.
Зарегистрируйтесь или войдите, чтобы иметь возможность оставить комментарий.