Package java.util.function
1. Commonly Used Functional Interfaces
Java SE 8 has added a lot of common functional interfaces, which can be used by the developer in lambda expressions instead of creating their own. They are defined in java.util.function package.
The most commonly used functional interfaces are:
Interface | Function Descriptor | Description |
---|---|---|
Consumer<T> | T -> void | Represents an operation that accepts a single input argument and returns no result. |
Function<T, R> | T -> R | Represents a function that accepts one argument and produces a result. |
Predicate<T> | T -> boolean | Represents a predicate (boolean-valued function) of one argument. |
Supplier<T> | () -> T | Represents a supplier of results. |
UnaryOperator<T> | T -> T | Represents an operation on a single operand that produces a result of the same type as its operand. |
2. Binary Specializations
The Predicate, Consumer, Function, and UnaryOperator functional interfaces represent an operation that takes one argument. But there are versions of these interfaces that take two arguments, called. These are the binary versions. They have the same semantics, the only difference is the number of arguments. Note, there is no binary version of the Supplier. This is because a Supplier takes no arguments.
The binary versions of the functional interfaces are:
Interface | Function Descriptor | Description |
---|---|---|
BiConsumer<T, U> | (T, U) -> void | Represents an operation that accepts two input arguments and returns no result. |
BiFunction<T, U, R> | (T, U) -> R | Represents a function that accepts two arguments and produces a result. |
BiPredicate<T, U> | (T, U) -> boolean | Represents a predicate (boolean-valued function) of two arguments. |
BinaryOperator<T> | (T, T) -> T | Represents an operation upon two operands of the same type, producing a result of the same type as the operands. |
3. Primitive Specializations
Every Java type is either a reference type (for example, String, Long, Object, List) or a primitive type (for example, int, long, char, boolean). But generic parameters (for example, the T in Supplier) can be bound only to reference types.
Java has a mechanism to convert a primitive type into a corresponding reference type (boxing) and a mechanism to convert a reference type into a corresponding primitive type (unboxing). An autoboxing mechanism facilitates boxing and unboxing operations for developers.
But this comes with a performance cost. Boxed values are essentially a wrapper around primitive types and are stored on the heap. Therefore, boxed values use more memory and require additional memory lookups to fetch the wrapped primitive value. Java 8 brings a specialized version of the functional interfaces in order to avoid autoboxing operations when the inputs or outputs are primitives. For example, in the following code, using an IntConsumer avoids a boxing operation of the value:
@FunctionalInterface
public interface IntConsumer {
void accept(int value);
...
}
IntConsumer ic = i -> System.out.println(++i);
ic.accept(8);
ic.accept(9);
The names of functional interfaces that have a specialization for the input type parameter are preceded by the appropriate primitive type, for example, IntFunction, DoublePredicate, LongBinaryOperator, IntConsumer. The Function interface has also variants for the output type parameter: ToIntFunction, ToDoubleFunction.
3.1. Predicate<T> Primitive Specializations
Function Descriptor: T -> boolean
Interface | Description |
---|---|
IntPredicate | Represents a predicate (boolean-valued function) of one int-valued argument. |
LongPredicate | Represents a predicate (boolean-valued function) of one long -valued argument. |
DoublePredicate | Represents a predicate (boolean-valued function) of one double -valued argument. |
3.2. Consumer<T> Primitive Specializations
Function Descriptor: T -> void
Interface | Description |
---|---|
IntConsumer | Represents an operation that accepts a single int-valued argument and returns no result. |
LongConsumer | Represents an operation that accepts a single long -valued argument and returns no result. |
DoubleConsumer | Represents an operation that accepts a single double -valued argument and returns no result. |
3.3. Function<T, R> Primitive Specializations
Function Descriptor: T -> R
The Function interface has variants not only for the input type parameters but for output and input/output as well. They are divided into three separate tables:
Interface | Description |
---|---|
IntFunction<R> | Represents a function that accepts an int-valued argument and produces a result. |
LongFunction<R> | Represents a function that accepts a long-valued argument and produces a result. |
DoubleFunction<R> | Represents a function that accepts a double-valued argument and produces a result. |
Interface | Description |
---|---|
ToIntFunction<T> | Represents a function that produces an int-valued result. |
ToDoubleFunction<T> | Represents a function that produces a double-valued result. |
ToLongFunction<T> | Represents a function that produces a long-valued result. |
Interface | Description |
---|---|
IntToDoubleFunction | Represents a function that accepts an int-valued argument and produces a double-valued result. |
IntToLongFunction | Represents a function that accepts an int-valued argument and produces a long-valued result. |
LongToDoubleFunction | Represents a function that accepts a long-valued argument and produces a double-valued result. |
LongToIntFunction | Represents a function that accepts a long-valued argument and produces an int-valued result. |
DoubleToIntFunction | Represents a function that accepts a double-valued argument and produces an int-valued result. |
DoubleToLongFunction | Represents a function that accepts a double-valued argument and produces a long-valued result. |
3.4. Supplier<T> Primitive Specializations
Function Descriptor: () -> T
Interface | Description |
---|---|
BooleanSupplier | Represents a supplier of boolean-valued results. |
IntSupplier | Represents a supplier of int-valued results. |
LongSupplier | Represents a supplier of long -valued results. |
DoubleSupplier | Represents a supplier of double -valued results. |
3.5. UnaryOperator<T> Primitive Specializations
Function Descriptor: T -> T
Interface | Description |
---|---|
IntUnaryOperator | Represents an operation on a single int-valued operand that produces an int-valued result. |
LongUnaryOperator | Represents an operation on a single long -valued operand that produces a long -valued result. |
DoubleUnaryOperator | Represents an operation on a single double -valued operand that produces a double -valued result. |
3.6. BinaryOperator<T> Primitive Specializations
Function Descriptor: (T, T) -> T
Interface | Description |
---|---|
IntBinaryOperator | Represents an operation upon two int -valued operands and producing an int -valued result. |
LongBinaryOperator | Represents an operation upon two long-valued operands and producing a long-valued result. |
DoubleBinaryOperator | Represents an operation upon two double-valued operands and producing a double-valued result. |
3.7. BiConsumer<T, U> Primitive Specializations
Function Descriptor: (T, U) -> void
Interface | Description |
---|---|
ObjIntConsumer<T> | Represents an operation that accepts an object-valued and an int-valued argument, and returns no result. |
ObjLongConsumer<T> | Represents an operation that accepts an object-valued and a long-valued argument, and returns no result. |
ObjDoubleConsumer<T> | Represents an operation that accepts an object-valued and a double -valued argument, and returns no result. |
3.8. BiFunction<T, U, R> Primitive Specializations
Function Descriptor: (T, U) -> R
Interface | Description |
---|---|
ToIntBiFunction<T, U> | Represents a function that accepts two arguments and produces an int-valued result. |
ToLongBiFunction<T, U> | Represents a function that accepts two arguments and produces a long-valued result. |
ToDoubleBiFunction<T, U> | Represents a function that accepts two arguments and produces a double-valued result. |
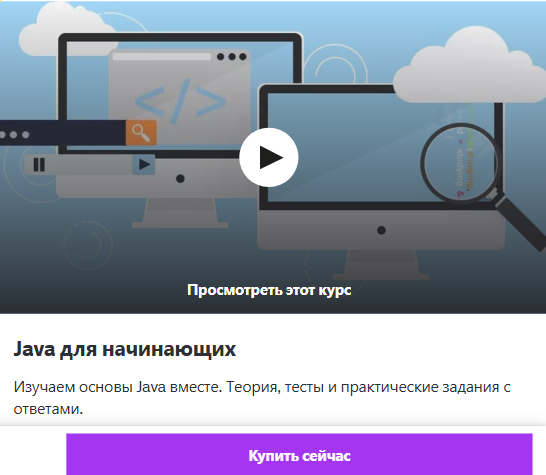
Please log in or register to have a possibility to add comment.