Functional Interface Function
Function<T, R> is a built-in functional interface included in Java SE 8 in the java.util.function package. The main purpose of this interface is mapping scenarios - when an object of one type is taken as input, and it is converted (or mapped) to another type. The most common case for using the Function interface is in streams, where the map function of a stream accepts an instance of a Function to convert the stream of one type to a stream of another type.
Example 1. java.util.function.Function Interface
The definition of the interface is shown in the example:
@FunctionalInterface
public interface Function<T, R> {
R apply(T t);
...
}
Function descriptor of the interface is:
T -> R
Example 2. Use of java.util.function.Function interface in Lambda Expression
Let's look at the usage of java.util.function.Function interface. There are Exam objects, which should be converted to String:
public class Exam {
private String name;
private String version;
public Exam(String name, String version) {
this.name = name;
this.version = version;
}
public String getName() {
return name;
}
public String getVersion() {
return version;
}
}
Function<Exam, String> converter = e -> e.getName() + " " + e.getVersion();
System.out.println(converter.apply(new Exam("OCPJP", "8")));
System.out.println(converter.apply(new Exam("OCPJP", "7")));
System.out.println(converter.apply(new Exam("OCJP", "6")));
The output is:
OCPJP 8
OCPJP 7
OCJP 6
The Function interface also has the following default methods:
default <V> Function<V,R> compose(
Function<? super V,? extends T< before)
default <V> Function<T,V> andThen(
Function<? super R,? extends V> after)
The compose method applies the function represented by the parameter first, and its result serves as the input to the other function. The andThen method first applies the function that calls the method, and its result acts as the input of the function represented by the parameter.
Let's look at the example of using these two methods:
Example 3. Use of andThen and compose methods of java.util.function.Function interface
Function<String, String> f1 = s -> s + "-f1-";
Function<String, String> f2 = s -> s + "-f2-";
Function<String, String> f3 = s -> s + "-f3-";
System.out.println(f1.andThen(f3).compose(f2).apply("Compose"));
System.out.println(f1.andThen(f2).andThen(f3).apply("AndThen"));
The output is:
Compose-f2--f1--f3-
AndThen-f1--f2--f3-
The Function interface has a static method, which returns a function that always returns its input argument:
static <T> Function<T, T> identity()
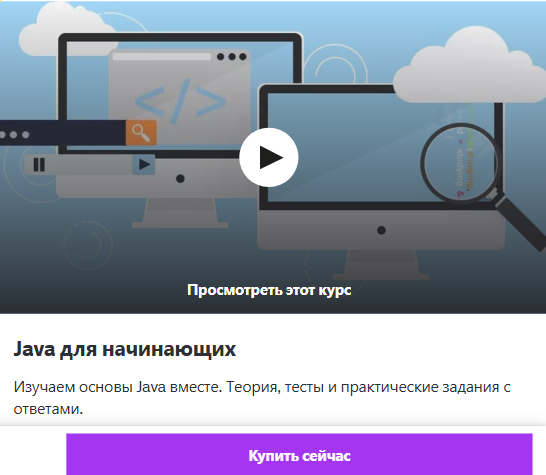
Please log in or register to have a possibility to add comment.