If Statement in Java: Explained
The if statement is one of the most frequently used conditional operators in Java. It's essential for directing program execution based on conditions.
1. If Statement in Java
The general syntax of the if
statement:
if (booleanExpression) {
System.out.println("In the if block");
}
Here, booleanExpression must return a value of type boolean or Boolean. If it evaluates to true, the code inside the curly braces executes. Otherwise, the block is skipped.
Example:
public class IfExample {
public static void main(String[] args) {
int a = 1;
if (a < 10) {
System.out.println("In the if block");
}
}
}
2. If-Else Statement
The if
statement can include an else
block, which executes if the booleanExpression
evaluates to false
.
if (booleanExpression) {
System.out.println("Inside if block");
} else {
System.out.println("Inside else block");
}
Example:
public class IfElseExample {
public static void main(String[] args) {
int a = 1;
if (a == 10) {
System.out.println("In the if block");
} else {
System.out.println("In the else block");
}
}
}
3. If-Else-If Statement
The if-else-if
ladder is a special case of the if-else
structure. It allows multiple conditions to be tested sequentially. Once a condition is met, the rest are skipped.
Example:
public class IfElseExample1 {
public static void main(String[] args) {
int month = 4; // April
String season;
if (month == 12 || month == 1 || month == 2) {
season = "Winter";
} else if (month == 3 || month == 4 || month == 5) {
season = "Spring";
} else if (month == 6 || month == 7 || month == 8) {
season = "Summer";
} else if (month == 9 || month == 10 || month == 11) {
season = "Autumn";
} else {
season = "Not a Month";
}
System.out.println("April is in the " + season + ".");
}
}
Output:April is in the Spring.
Best Practices:
- Always use curly braces
{}
even for single-line blocks to enhance code readability. - Ensure logical expressions are clear to avoid confusion.
By mastering conditional statements like if
, if-else
, and if-else-if
, you gain the ability to control the flow of your Java programs effectively.
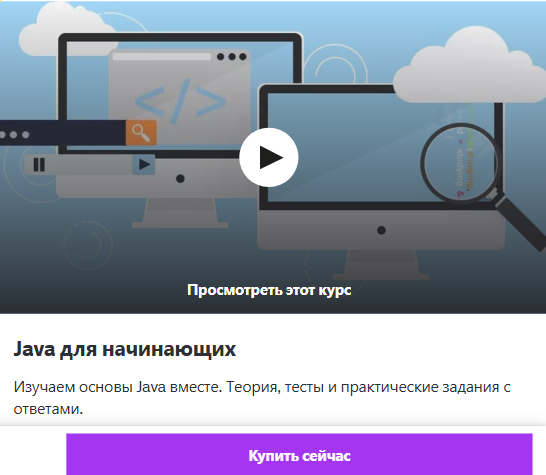
Please log in or register to have a possibility to add comment.