Switch-Case Statement in Java 14
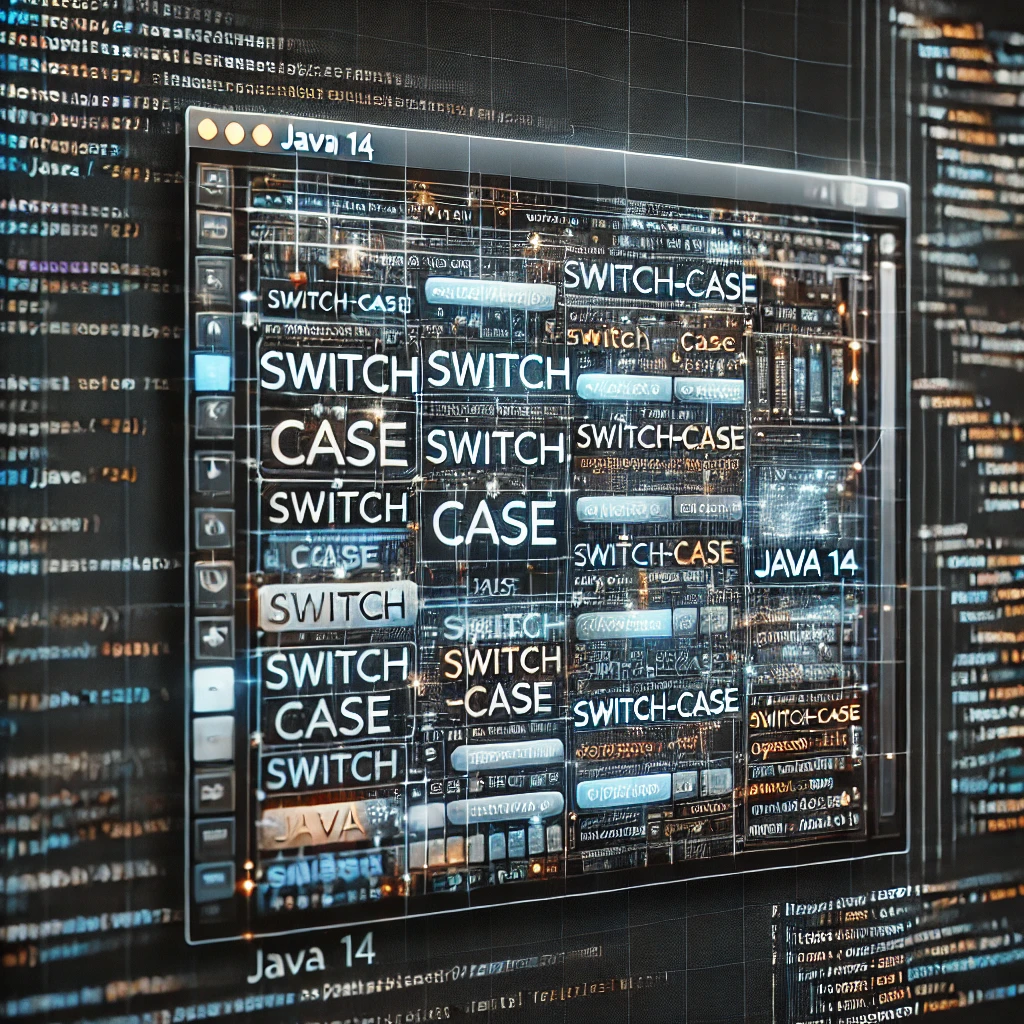
The switch statement in Java is a conditional construct allowing comparison of a variable against a list of values. Java 14 introduced significant changes to its syntax, enhancing readability and functionality.
1. switch Before Java 14
Prior to Java 14, a switch block followed a specific syntax:
switch (expression) {
case value1:
// Code block
break;
case value2:
// Code block
break;
default:
// Default block
}
Example:
public class TrafficLight {
public static void main(String[] args) {
int x = 3;
switch (x) {
case 1:
System.out.println("Green");
break;
case 2:
System.out.println("Yellow");
break;
case 3:
System.out.println("Red");
break;
default:
System.out.println("Invalid value");
break;
}
}
}
Switch expressions can only check for equality. Logical operators like >=
or <=
are not allowed. Additionally, switch can handle types like char
, byte
, short
, int
, enum
(Java 6+), or String
(Java 7+).
For multiple case values, you can specify a single block, as shown in the following example:
public class SwitchExample1 {
public static void main(String[] args) {
int month = 4;
String season;
switch (month) {
case 12:
case 1:
case 2:
season = "Winter";
break;
case 3:
case 4:
case 5:
season = "Spring";
break;
case 6:
case 7:
case 8:
season = "Summer";
break;
case 9:
case 10:
case 11:
season = "Autumn";
break;
default:
season = "Not a Month";
}
System.out.println("April is in the " + season + ".");
}
}
If the expression in a switch statement has a type smaller than int
(such as byte
, short
, or char
), the case
constant must match that type. For example, the following code will not compile:
byte number = 2;
switch (number) {
case 13:
case 129://compiler error
}
It is also not allowed to use multiple case
constants with the same value. The following code block will not compile:
int number = 90;
switch (number) {
case 50:
System.out.println("50");
case 50:
System.out.println("50"); //compile error
case 140:
System.out.println("140");
default:
System.out.println("default");
}
A switch expression can contain values of type Character
, Byte
, Short
, and Integer
:
switch (new Integer(9)) {
case 9:
System.out.println("9");
}
Case constants are evaluated from top to bottom, and the first matching case constant in the switch serves as the entry point. The corresponding block and all subsequent blocks will be executed. For example:
public class SwitchExample2 {
public static void main(String[] args) {
String str = "potato";
switch (str) {
case "tomato":
System.out.print("tomato ");
case "potato":
System.out.print("potato ");
case "cucumber":
System.out.print("cucumber ");
default:
System.out.println("any");
}
}
}
Result:
potato cucumber any
To exit a switch statement, use the break keyword. For example, let's modify the previous example so that only one word is displayed:
Vegetable p = Vegetable.potato;
switch (p) {
case tomato:
System.out.print("tomato ");
break;
case potato:
System.out.print("potato ");
break;
case cucumber:
System.out.print("cucumber ");
break;
default:
System.out.println("any");
}
The result:
potato
The default section in a switch handles all values not explicitly specified in one of the case sections. The default section can be placed anywhere, not necessarily at the end:
public class SwitchExample3 {
public static void main(String[] args) {
int z = 8;
switch (z) {
case 1:
System.out.println("Fall to one");
default:
System.out.println("default");
case 3:
System.out.println("Fall to three");
case 4:
System.out.println("Fall to four");
}
}
}
The default section in a switch handles all values not explicitly specified in one of the case sections. The default section can be placed anywhere, not necessarily at the end:
String exam = null;
switch (exam) {
case "OCPJP 7":
System.out.print(exam + ": 1Z0-804");
break;
case "OCPJP 8":
System.out.print(exam + ": 1Z0-809");
break;
default:
System.out.print(exam + ": ----");
break;
}
The following example demonstrates the use of switch with an enum:
CoffeeSize coffeeSize = CoffeeSize.BIG;
double price = switch (coffeeSize) {
case BIG:
yield 2;
case HUGE:
yield 3;
case OVERWHELMING:
yield 4;
};
Suppose we have an enumeration CoffeeSize with only three values: BIG, HUGE, and OVERWHELMING. If all the enumeration values are covered in the switch, the default block can be omitted.
2. Switch in Java 14
Now let's move on to the changes introduced in Java 14. Previously, the switch was called a statement, but now it's referred to as a switch expression. Switch expressions first appeared not in Java 14, but in Java 12 as a preview version. In Java 13, they were improved, and in Java 14, they became part of the main release.
The switch expression significantly simplifies the code and makes it more readable with the use of arrow case.
Let's take a look at a switch example in Java 14:
public static void main(String[] args) {
int x = 2;
switch (x) {
case 1 -> System.out.println("Green");
case 2 -> System.out.println("Yellow");
case 3 -> {
System.out.println("Red");
System.out.println("You cannot move");
}
default -> System.out.println("Invalid number");
}
}
The result:
Yellow
Notice how things have changed compared to the previous version. Now, instead of a colon, an arrow (arrow case) is used. After the arrow, there can either be a single line of code or a block of code. If it's a block, curly braces are used.
Another change in Java 14 is that only one block is executed at a time. This means that the fall-through behavior, which was present in the old version of switch, no longer occurs. As a result, there is no need to write a break
for each block of code, as was required in the old version.
Often, the switch not only needs to execute a block of code but also return a value:
public class SwitchExample2 {
public static void main(String[] args) {
int month = 4;
String season = switch (month) {
case 12, 1, 2 -> "Winter";
case 3, 4, 5 -> "Spring";
case 6, 7, 8 -> "Summer";
case 9, 10, 11 -> "Autumn";
default -> "Not a Month";
};
System.out.println("April is in the " + season + ".");
}
}
In Java 14, the reserved keyword yield
was introduced, which simplifies the logic for returning a result:
public class SwitchExample1 {
public static void main(String[] args) {
int month = 4;
String season = switch (month) {
case 12, 1, 2:
yield "Winter";
case 3, 4, 5:
yield "Spring";
case 6, 7, 8:
yield "Summer";
case 9, 10, 11:
yield "Autumn";
default:
yield "Not a Month";
};
System.out.println("April is in the " + season + ".");
}
}
In each case, you can use the reserved keyword yield
, which returns a value.
The difference between a reserved word and a keyword is that keywords cannot be used as identifiers. However, reserved words can be used as identifiers outside of their context. Outside the switch block, you can create a variable with the name yield
, and there will be no compilation error:
public static void main(String[] args) {
int month = 4;
String season = switch (month) {
case 12, 1, 2:
yield "Winter";
default:
yield "Not a Month";
};
int yield = 6 ;
}
However, you cannot create a variable with a keyword, such as case
, even outside of the switch block. It will result in a compilation error.
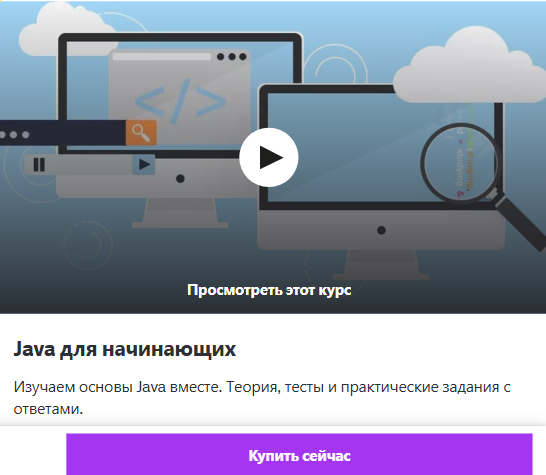
Please log in or register to have a possibility to add comment.