Functional Interface UnaryOperator
The UnaryOperator is a built-in functional interface included in Java SE 8 in the java.util.function package, that extends java.util.function.Function. It is used to work on a single operand, and it returns the same type as an operand. The UnaryOperator is used as a lambda expression to pass as an argument.
Example 1. java.util.function.UnaryOperator Interface
The definition of the interface is shown in the example:
@FunctionalInterface
public interface UnaryOperator<T> extends Function<T, T> {
...
}
Function descriptor of the interface is:
T -> T
Example 2. Usage of java.util.function.UnaryOperator Interface
Let's look at the simple example:
UnaryOperator<String> uo = s -> s.toUpperCase();
System.out.print(uo.apply("Ocpjp 8"));
This example can be rewritten with a method reference:
UnaryOperator<String> uo = String::toUpperCase;
System.out.print(uo.apply("Ocpjp 8"));
The UnaryOperator interface inherits the default methods of the Function interface:
default <V> Function<V,R> compose(
Function<? super V,? extends T> before)
default <V> Function<T,V> andThen(
Function<? super R,? extends V> after)
And defines the static method identity() for the interface:
static <T> UnaryOperator<T> identity()
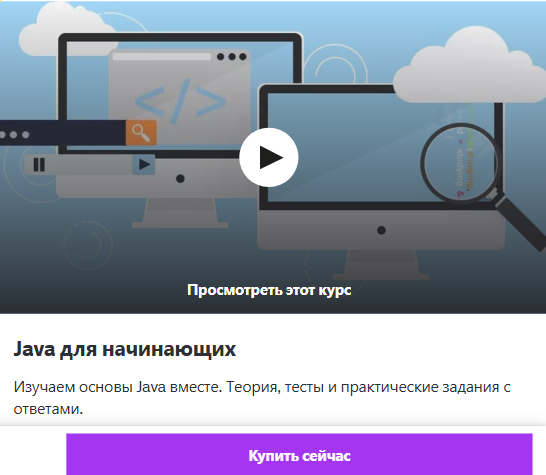
Please log in or register to have a possibility to add comment.