JAX-RS Exception Handling
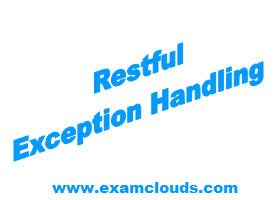
The JAX-RS provides two possibilities for restful web services exception handling - WebApplicationException and mapping exceptions to responses.
1. WebApplicationException
The JAX-RS has a special RuntimeException class - WebApplicationException, which gives the possibility to abort the JAX-RS service method. It can take an HTTP status code or even a Response object as one of its constructor parameters.
Example 1.1. Throw WebApplicationException from resource method
If the exam is null, it is created a response with the help of ResponseBuilder. An HTTP response code is set to NOT_FOUND. A constructed response is passed to the WebApplicationException:
@GET
@Path("{id}")
public Exam get(@PathParam("id") int id) {
Exam exam = foundExam(id);
if (exam == null) {
Response.ResponseBuilder builder = Response.status(Response.Status.NOT_FOUND);
builder.type("text/html");
builder.entity("<h1>Exam Not Found</h1>");
throw new WebApplicationException(builder.build());
}
return exam;
}
Example 1.2. Throw NotFoundException
The previous example can be simplified. There is a special NotFoundException, which builds an HTTP response with the 404 status code and an optional message as the body of the response. The NotFoundException extends WebApplicationException.
@GET
@Path("{id}")
public Exam get(@PathParam("id") int id) {
Exam exam = foundExam(id);
if (exam == null) {
throw new NotFoundException("Exam Not Found");
}
return exam;
}
2. Mapping Exceptions to Responses
JAX-RS provides a possibility to map an existing exception to response as well. It is required to implement ExceptionMapper<E extends Throwable> interface.
Example 2.1. Mapping generic exceptions to a response
The example maps ExamNotFoundException to an HTTP 404 (Not Found) response. The ExamNotFoundMapper class is annotated with @Provider to declare the class to be of interest to the JAX-RS runtime. When an application throws an ExamNotFoundException, the ExamNotFoundMapper.toResponse method will be called.
public class ExamNotFoundException extends RuntimeException {
public ExamNotFoundException(String message) {
super(message);
}
}
@Provider
public class ExamNotFoundMapper implements ExceptionMapper<ExamNotFoundException> {
public Response toResponse(ExamNotFoundException exception) {
return Response.status(Response.Status.NOT_FOUND).
entity(exception.getMessage()).
type("text/plain").
build();
}
}
@GET
@Path("{id}")
public Exam get(@PathParam("id") int id) {
Exam exam = foundExam(id);
if (exam == null) {
throw new ExamNotFoundException("Exam is not Found");
}
return exam;
}
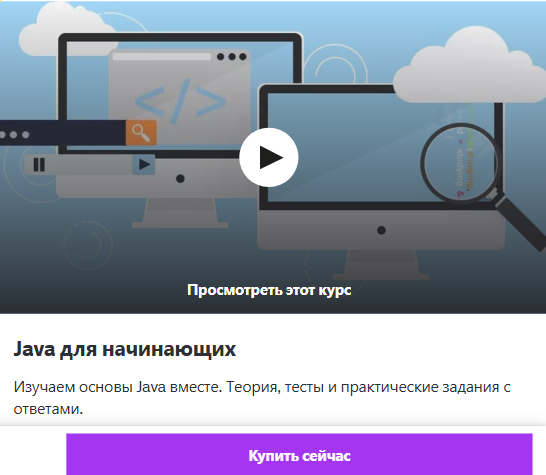
Зарегистрируйтесь или войдите, чтобы иметь возможность оставить комментарий.