Method System.arraycopy()
The System.arraycopy() method in Java allows you to copy a portion of one array into another quickly and efficiently.
Let's look at an example that copies elements 2, 3, and 4 from the arraySource
into the arrayDestination
array:
import java.util.Arrays;
public class ArrayCopyExample1 {
public static void main(String[] args) {
int[] arraySource = {1, 2, 3, 4, 5, 6};
int[] arrayDestination = {0, 0, 0, 0, 0, 0, 0, 0};
System.out.println("arraySource: " + Arrays.toString(arraySource));
System.out.println("arrayDestination: " + Arrays.toString(arrayDestination));
System.arraycopy(arraySource, 1, arrayDestination, 2, 3);
System.out.println("arrayDestination after arrayCopy: " + Arrays.toString(arrayDestination));
}
}
Output:
arraySource: [1, 2, 3, 4, 5, 6]
arrayDestination: [0, 0, 0, 0, 0, 0, 0, 0]
arrayDestination after arrayCopy: [0, 0, 2, 3, 4, 0, 0, 0]
Note: The syntax of the method is:
System.arraycopy(src, srcPos, dest, destPos, length);
Where:
src
– source arraysrcPos
– starting position in the source arraydest
– destination arraydestPos
– starting position in the destination arraylength
– number of elements to copy
You can also copy elements within the same array, including overlapping regions. This is often useful for array manipulation or shifting values:
import java.util.Arrays;
public class ArrayCopyExample2 {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5, 6, 7, 8};
System.out.println(Arrays.toString(array));
System.arraycopy(array, 1, array, 3, 3);
System.out.println(Arrays.toString(array));
}
}
Output:
[1, 2, 3, 4, 5, 6, 7, 8]
[1, 2, 3, 2, 3, 4, 7, 8]
The System.arraycopy()
method performs a shallow copy, meaning that if you copy arrays of objects, only the references are copied, not the actual object contents.
This method is more efficient than manual copying using loops and is widely used in performance-critical applications, such as data buffers and memory manipulation.
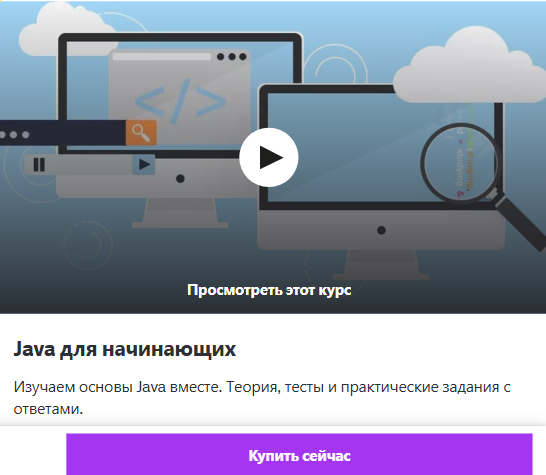
Please log in or register to have a possibility to add comment.