Functional Interface Supplier
Supplier<T> is a built-in functional interface included in Java SE 8 in the java.util.function package. The Supplier can be used in cases when there is no input, but output is expected.
Example 1. java.util.function.Supplier Interface
The definition of the interface is shown in the example:
@FunctionalInterface
public interface Supplier<T> {
T get();
}
Function descriptor of the interface is:
() -> T
Example 2. Usage of java.util.function.Supplier Interface
Let's look at the simplest example of Supplier interface usage, where it is just returned an object instance:
public class Animal {
public void move() {
System.out.println("Move");
}
@Override
public String toString() {
return "Some Animal";
}
}
public class TestAnimal {
public static void main(String args[]) {
Supplier<Animal> s = () -> new Animal();
System.out.println(s.get());
}
}
Example 3. Usage of java.util.function.Supplier Interface with Method Overriding
This example illustrates how the Supplier interface can be used with method overriding. A class Bird extends class Animal and overrides its move() method. The static method moveAnimal() invokes the move() method of the instance of Animal or Bird. To call moveAnimal(), an implementation of Supplier interface should be passed:
public class Animal {
public void move() {
System.out.println("Move");
}
}
public class Bird extends Animal {
@Override
public void move(){
System.out.println("Fly");
}
}
public class TestAnimal {
public static void main(String args[]) {
moveAnimal(() -> new Animal());
moveAnimal(() -> new Bird());
}
public static void moveAnimal(Supplier<Animal> supplier) {
Animal animal = supplier.get();
animal.move();
}
}
Example 4. Usage of java.util.function.Supplier Interface with Method Reference
We can rewrite the main method of the previous example to use method reference:
public static void main(String args[]) {
moveAnimal(Animal::new);
moveAnimal(Bird::new);
}
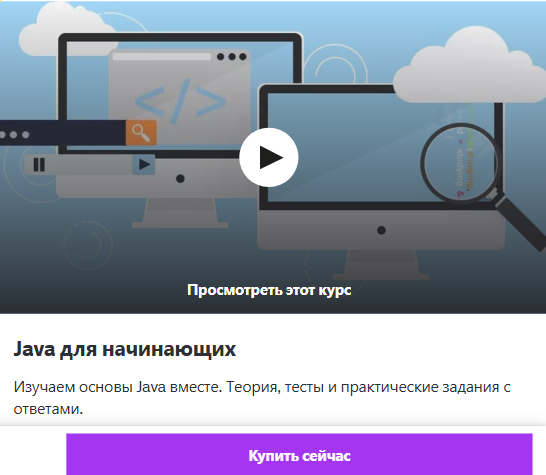
Please log in or register to have a possibility to add comment.